通过这个代码可以实现软件的快捷方式的调用:
class HotKey
{
//如果函数执行成功,返回值不为0。
//如果函数执行失败,返回值为0。要得到扩展错误信息,调用GetLastError。
[DllImport("user32.dll", SetLastError = true)]
public static extern bool RegisterHotKey(
IntPtr hWnd, //要定义热键的窗口的句柄
int id, //定义热键ID(不能与其它ID重复)
KeyModifiers fsModifiers, //标识热键是否在按Alt、Ctrl、Shift、Windows等键时才会生效
Keys vk //定义热键的内容
);
[DllImport("user32.dll", SetLastError = true)]
public static extern bool UnregisterHotKey(
IntPtr hWnd, //要取消热键的窗口的句柄
int id //要取消热键的ID
);
//定义了辅助键的名称(将数字转变为字符以便于记忆,也可去除此枚举而直接使用数值)
[Flags()]
public enum KeyModifiers
{
None = 0,
Alt = 1,
Ctrl = 2,
Shift = 4,
WindowsKey = 8
}
}
在form中, 启用Form1_Activated 和 Form1_Deactivate
private void Form1_Activated(object sender, EventArgs e)
{
HotKey.RegisterHotKey(Handle, 100, HotKey.KeyModifiers.Ctrl, Keys.A);
HotKey.RegisterHotKey(Handle, 101, HotKey.KeyModifiers.Ctrl, Keys.B);
HotKey.RegisterHotKey(Handle, 102, HotKey.KeyModifiers.Ctrl, Keys.C);
HotKey.RegisterHotKey(Handle, 103, HotKey.KeyModifiers.Ctrl, Keys.D);
//自行更改快捷键按钮代码
}
private void Form1_Deactivate(object sender, EventArgs e)
{
HotKey.UnregisterHotKey(Handle, 100);
HotKey.UnregisterHotKey(Handle, 101);
HotKey.UnregisterHotKey(Handle, 102);
HotKey.UnregisterHotKey(Handle, 103);
//自行补充自定义增加的快捷键
}
只用重写 wndProc:
protected override void WndProc(ref Message m)
{
const int WM_HOTKEY = 0x0312;
//按快捷键
switch (m.Msg)
{
case WM_HOTKEY:
switch (m.WParam.ToInt32())
{
case 100:
this.Text = "CTRL+A;";
break;
case 101:
this.Text = "CTRL+B;";
break;
default:
// MessageBox.Show("按下了"+ m.WParam.ToInt32().ToString());
break;
}
break;
}
base.WndProc(ref m);
}
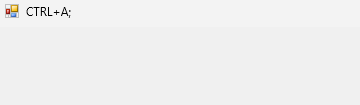
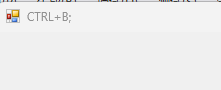
按下 ctrl + A,ctrl + B之后, 就能看到变化了。
该文章在 2024/10/14 12:21:48 编辑过