本文将详细介绍如何使用 C# 在 SQLite 数据库中执行 SELECT 操作。SELECT 操作是数据库查询中最常用和最重要的操作,用于从数据库中检索数据。
准备工作
首先,确保你的项目中已安装 System.Data.SQLite
NuGet 包。在你的 C# 文件顶部添加以下 using 语句:
using System;
using System.Data;
using System.Data.SQLite;
连接到数据库
在执行任何查询之前,我们需要建立与数据库的连接。以下是一个建立连接的辅助方法:
public static SQLiteConnection ConnectToDatabase(string dbPath)
{
try
{
SQLiteConnection connection = new SQLiteConnection($"Data Source={dbPath};Version=3;");
connection.Open();
return connection;
}
catch (Exception ex)
{
Console.WriteLine($"连接数据库时出错:{ex.Message}");
return null;
}
}
基本的 SELECT 操作
查询所有记录
以下是一个查询表中所有记录的方法:
public static void SelectAllRecords(SQLiteConnection connection, string tableName)
{
try
{
string sql = $"SELECT * FROM {tableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"查询所有记录时出错:{ex.Message}");
}
}
使用示例:
SelectAllRecords(connection, "Users");
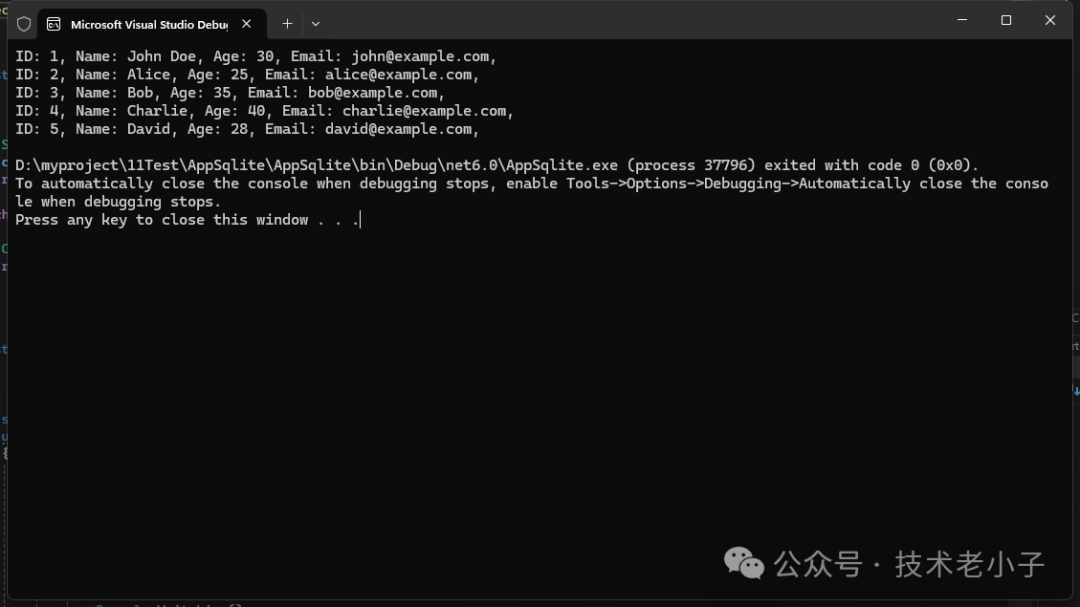
使用条件查询
以下是一个带条件的查询方法:
public static void SelectRecordsWithCondition(SQLiteConnection connection, string tableName, string condition)
{
try
{
string sql = $"SELECT * FROM {tableName} WHERE {condition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"条件查询记录时出错:{ex.Message}");
}
}
使用示例:
SelectRecordsWithCondition(connection, "Users", "Age > 30");
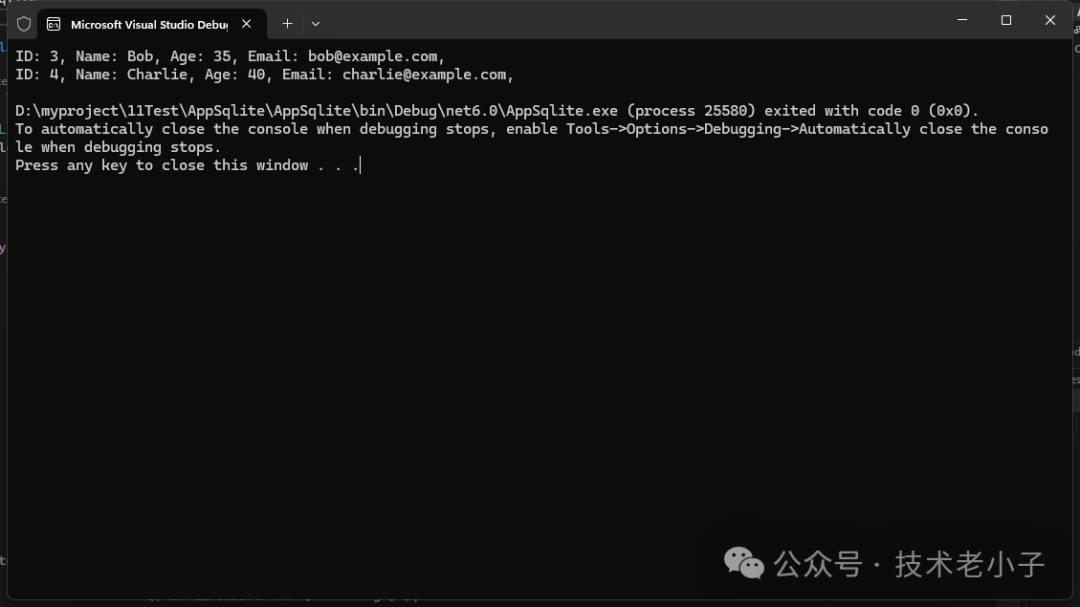
使用参数化查询
为了防止 SQL 注入攻击并提高性能,我们应该使用参数化查询:
public static void SelectRecordsWithParameters(SQLiteConnection connection, string tableName, string condition, Dictionary<string, object> parameters)
{
try
{
string sql = $"SELECT * FROM {tableName} WHERE {condition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
foreach (var param in parameters)
{
command.Parameters.AddWithValue(param.Key, param.Value);
}
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"参数化查询记录时出错:{ex.Message}");
}
}
使用示例:
var parameters = new Dictionary<string, object>
{
{ "@minAge", 25 },
{ "@maxAge", 40 }
};
SelectRecordsWithParameters(connection, "Users", "Age BETWEEN @minAge AND @maxAge", parameters);
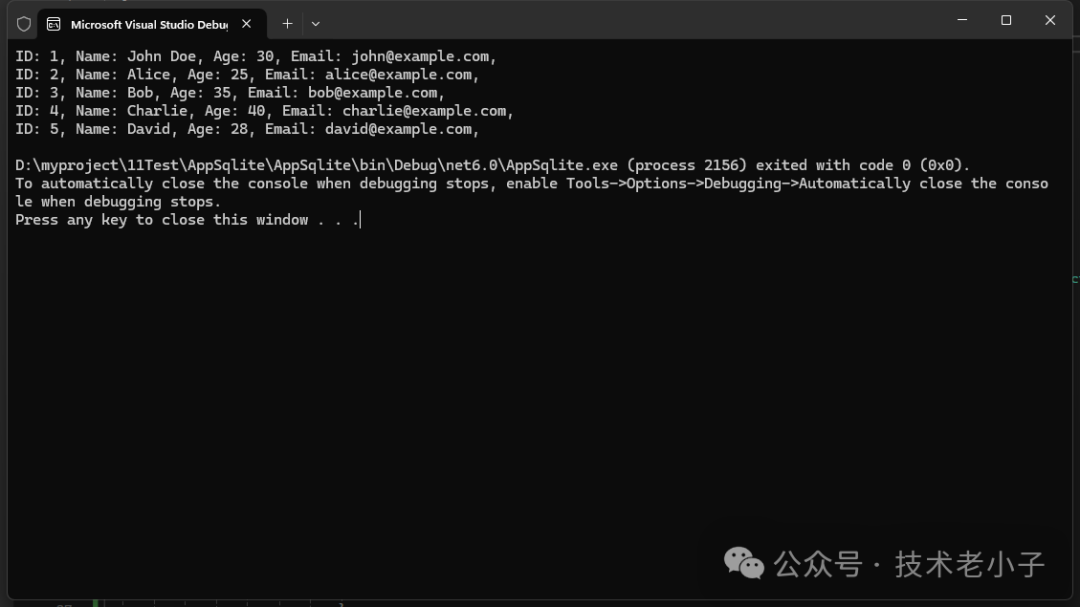
高级 SELECT 操作
使用聚合函数
SQLite 支持多种聚合函数,如 COUNT, AVG, SUM 等。以下是一个使用聚合函数的示例:
public static void SelectWithAggregation(SQLiteConnection connection, string tableName, string aggregation)
{
try
{
string sql = $"SELECT {aggregation} FROM {tableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
object result = command.ExecuteScalar();
Console.WriteLine($"聚合结果:{result}");
}
}
catch (Exception ex)
{
Console.WriteLine($"执行聚合查询时出错:{ex.Message}");
}
}
使用示例:
SelectWithAggregation(connection, "Users", "AVG(Age)");
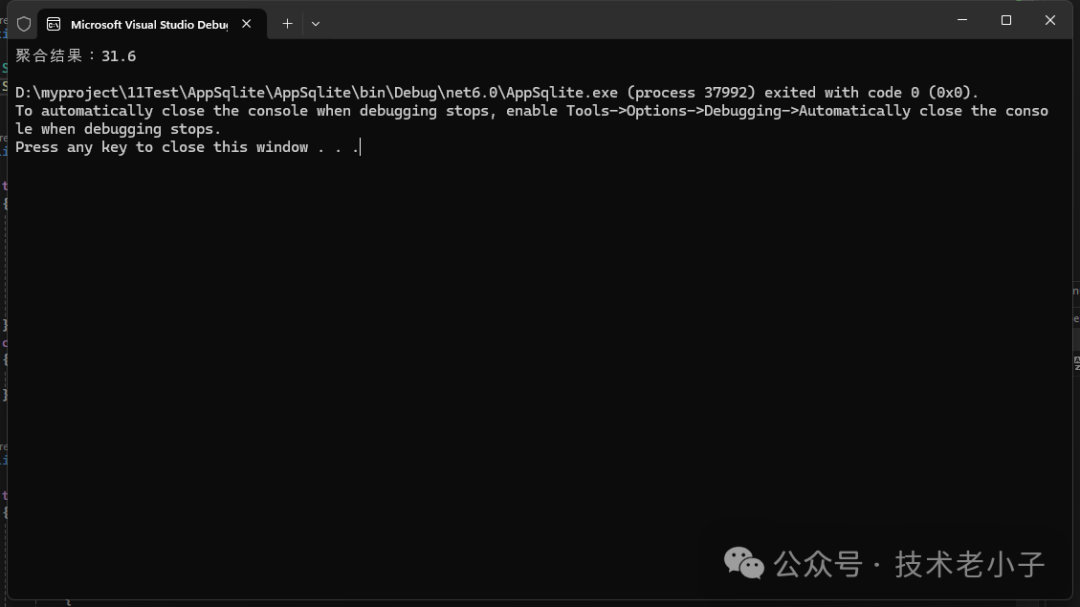
使用 ORDER BY 和 LIMIT
以下是一个带排序和限制结果数量的查询方法:
public static void SelectWithOrderAndLimit(SQLiteConnection connection, string tableName, string orderBy, int limit)
{
try
{
string sql = $"SELECT * FROM {tableName} ORDER BY {orderBy} LIMIT {limit}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"执行排序和限制查询时出错:{ex.Message}");
}
}
使用示例:
SelectWithOrderAndLimit(connection, "Users", "Age DESC", 5);
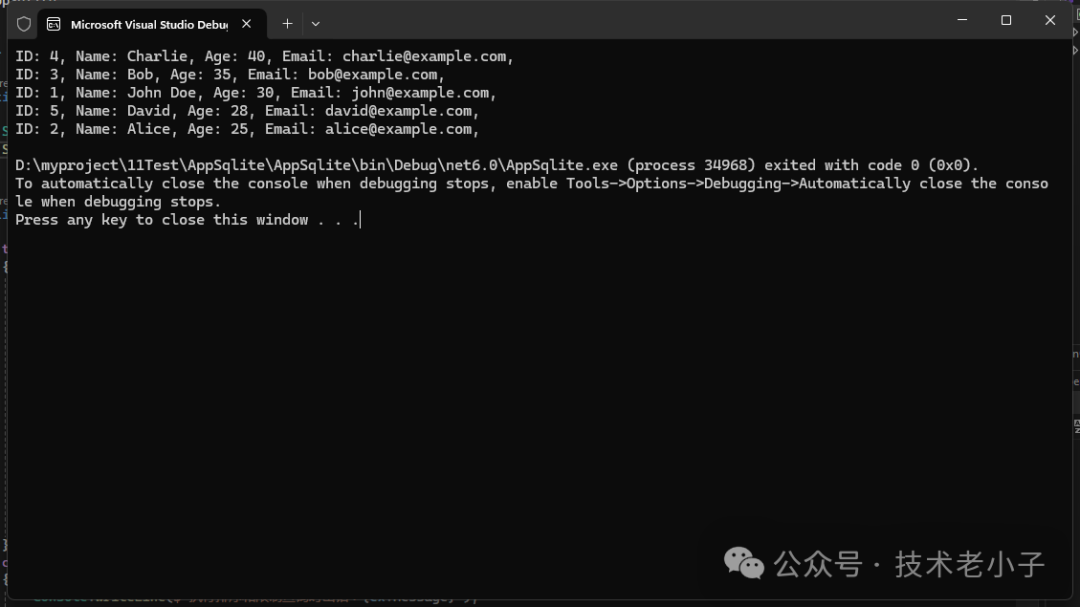
使用 JOIN
以下是一个使用 JOIN 的示例方法:
public static void SelectWithJoin(SQLiteConnection connection, string table1, string table2, string joinCondition)
{
try
{
string sql = $"SELECT * FROM {table1} INNER JOIN {table2} ON {joinCondition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"执行 JOIN 查询时出错:{ex.Message}");
}
}
使用示例:
SelectWithJoin(connection, "Users", "Orders", "Users.Id = Orders.UserId");
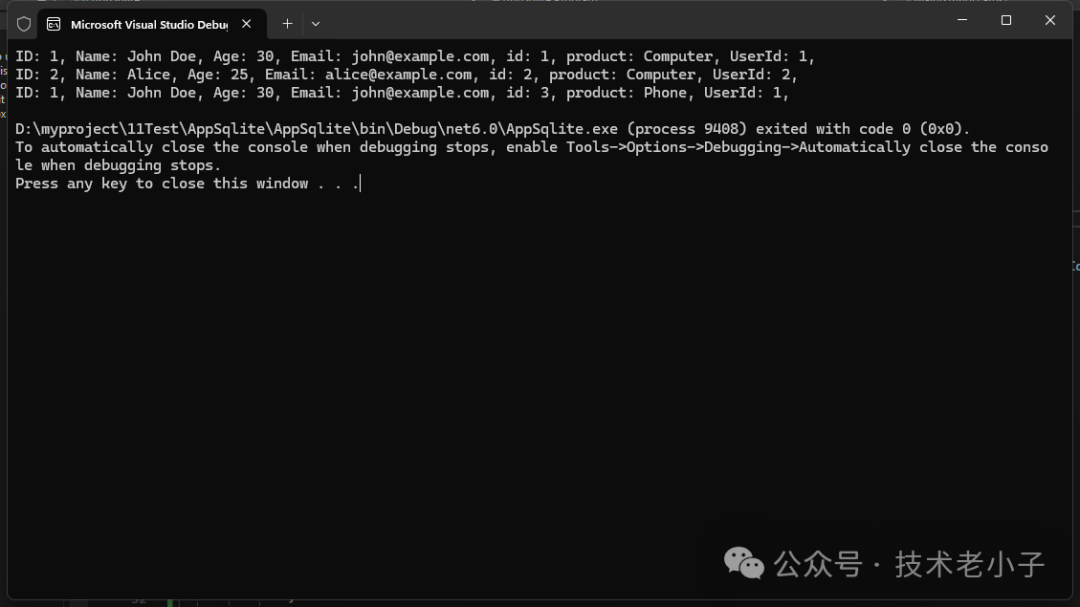
使用 DataTable 存储结果
对于需要在内存中处理查询结果的情况,我们可以使用 DataTable:
public static DataTable SelectToDataTable(SQLiteConnection connection, string sql)
{
try
{
DataTable dt = new DataTable();
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataAdapter adapter = new SQLiteDataAdapter(command))
{
adapter.Fill(dt);
}
}
return dt;
}
catch (Exception ex)
{
Console.WriteLine($"查询到 DataTable 时出错:{ex.Message}");
return null;
}
}
使用示例:
DataTable dt = SelectToDataTable(connection, "SELECT * FROM Users WHERE Age > 30");
foreach (DataRow row in dt.Rows)
{
foreach (DataColumn col in dt.Columns)
{
Console.Write($"{col.ColumnName}: {row[col]}, ");
}
Console.WriteLine();
}
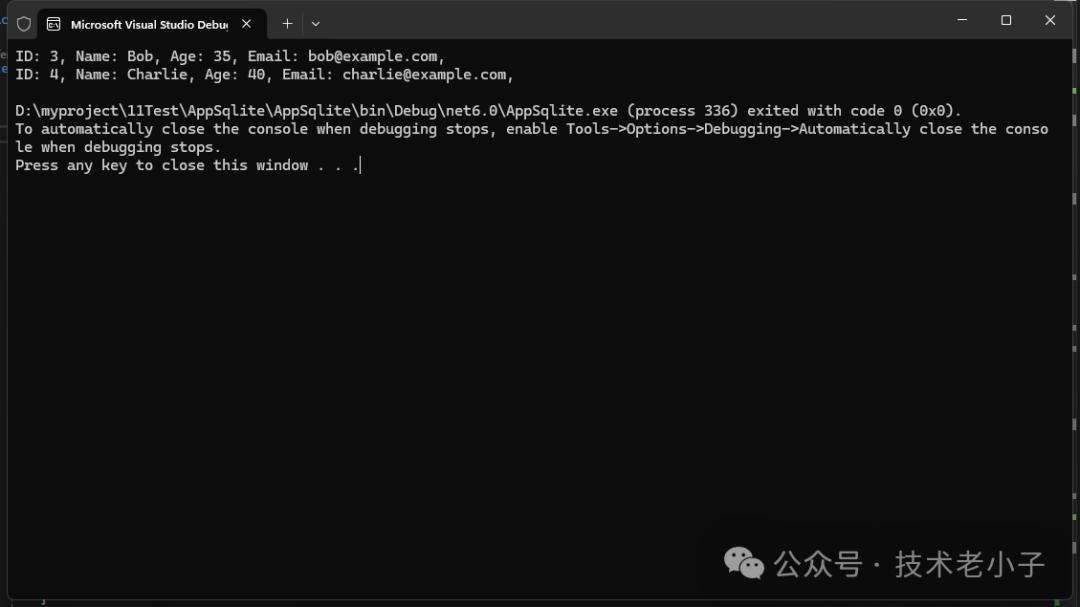
完整示例
以下是一个完整的示例,展示了如何使用上述所有方法:
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SQLite;
class Program
{
static void Main(string[] args)
{
string dbPath = "C:\\example.db";
SQLiteConnection connection = ConnectToDatabase(dbPath);
if (connection != null)
{
// 查询所有记录
Console.WriteLine("所有用户:");
SelectAllRecords(connection, "Users");
// 条件查询
Console.WriteLine("\n30岁以上的用户:");
SelectRecordsWithCondition(connection, "Users", "Age > 30");
// 参数化查询
Console.WriteLine("\n25到40岁的用户:");
var parameters = new Dictionary<string, object>
{
{ "@minAge", 25 },
{ "@maxAge", 40 }
};
SelectRecordsWithParameters(connection, "Users", "Age BETWEEN @minAge AND @maxAge", parameters);
// 聚合查询
Console.WriteLine("\n用户平均年龄:");
SelectWithAggregation(connection, "Users", "AVG(Age)");
// 排序和限制结果
Console.WriteLine("\n年龄最大的5个用户:");
SelectWithOrderAndLimit(connection, "Users", "Age DESC", 5);
// JOIN 查询
Console.WriteLine("\n用户及其订单:");
SelectWithJoin(connection, "Users", "Orders", "Users.Id = Orders.UserId");
// 查询到 DataTable
Console.WriteLine("\n30岁以上的用户(使用 DataTable):");
DataTable dt = SelectToDataTable(connection, "SELECT * FROM Users WHERE Age > 30");
foreach (DataRow row in dt.Rows)
{
foreach (DataColumn col in dt.Columns)
{
Console.Write($"{col.ColumnName}: {row[col]}, ");
}
Console.WriteLine();
}
// 关闭连接
connection.Close();
}
}
// 在这里实现所有上述方法
}
注意事项
始终使用参数化查询来防止 SQL 注入攻击。
对于大型结果集,考虑使用分页查询或流式处理来避免内存问题。
正确处理 NULL 值,特别是在使用聚合函数时。
在完成操作后,记得关闭数据库连接。
对于频繁执行的查询,可以考虑使用预处理语句(prepared statements)来提高性能。
结论
本文详细介绍了如何使用 C# 在 SQLite 数据库中执行各种 SELECT 操作,包括基本查询、条件查询、参数化查询、聚合查询、排序和限制结果、JOIN 操作以及使用 DataTable 存储结果。这些操作覆盖了大多数常见的数据检索场景。
该文章在 2024/10/22 12:24:35 编辑过